ChatGPT
10 Ways To Get More Done as a Web Developer with ChatGPT
As a web developer, your daily routine involves countless hours of coding, testing, and debugging. The complexity of the code can sometimes be overwhelming, and the search for solutions to problems can be time-consuming. Fortunately, with ChatGPT, you can take your productivity to the next level.
ChatGPT is an AI-powered conversational tool that can be accessed through various communication channels, including web-based chat interfaces. It can provide you with instant access to vast amounts of information, saving you time and effort.
Here are just some ways to get more done as a web developer with ChatGPT.
1. Generating Code
ChatGPT is most commonly used to generate code in different programming languages, including Python, Java, JavaScript, C++, and more.
Here’s an example prompt and response to using ChatGPT to generate code.
Prompt:
Fetch fake data in Javascript using Axios.
ChatGPT’s Response:
2. Explaining an Implementation
If you find it difficult to comprehend the exact purpose of a function, you can copy and paste it into ChatGPT and ask for an explanation. This is particularly useful if you want to quickly understand how the function works when commencing a project or traversing through unfamiliar codebases. Moreover, ChatGPT can be a mentor for people looking to learn programming skills with practical demonstrations.
Do you find it difficult to understand the concepts of bubble sort or depth-first search algorithms? Allow ChatGPT to assist you in the process by giving it an implementation and asking for a step-by-step explanation.
Here’s an example prompt and response to using ChatGPT to explain an implementation.
Prompt:
Can you explain this code?
def bubbleSort(arr):
n = len(arr)
# optimize code, so if the array is already sorted, it doesn’t need
# to go through the entire process
swapped = False
# Traverse through all array elements
for i in range(n-1):
# range(n) also work but outer loop will
# repeat one time more than needed.
# Last i elements are already in place
for j in range(0, n-i-1):
# traverse the array from 0 to n-i-1
# Swap if the element found is greater
# than the next element
if arr[j] > arr[j + 1]:
swapped = True
arr[j], arr[j + 1] = arr[j + 1], arr[j]
if not swapped:
# if we haven’t needed to make a single swap, we
# can just exit the main loop.
return
ChatGPT’s Response:
As you can see, ChatGPT’s answer is not generic at all. In fact, it used the same variable names that you provided in the code to give you this answer.
3. Translating Code
Web developers can also use ChatGPT to translate code from one programming language to another. You simply have to ask it to convert the [X] code to [Y] code, and then copy and paste the code snippet. However, we highly recommend using markdown syntax when you present the code.
Here’s an example prompt and response to using ChatGPT to translate code.
Prompt:
Convert this code to Python:
“`js
axios.get(“…”)
.then(data => console.log(data))
.catch(err => console.log(err))
“`
ChatGPT’s Response:
4. Improving Code Quality and Enforcing Good Practices
As a web developer, you must adhere to a set of standards and good practices when you write code. One such good practice is to validate input data when submitting it through an API endpoint.
Here’s an example prompt and response to using ChatGPT to improve code quality and enforce good practices.
Prompt:
Can you examine this code and give me pointers as to how to improve it?
from flask import Flask, Blueprint, request, jsonify
from flask_cors import CORS
app = Flask(__name__)
CORS(app)
api = Blueprint(‘api’, __name__)
@api.route(‘/submit’, methods=[‘POST’])
def handle_submit():
if request.method == “POST”:
first_name = request.form[‘firstName’]
last_name = request.form[‘lastName’]
job = request.form[‘job’]
print(f’first name : {first_name}’)
print(f’last name : {last_name}’)
print(f’job : {job}’)
# do your processing logic here.
return jsonify({
“firstName”: first_name,
“lastName”: last_name,
“job”: job
})
app.register_blueprint(api, url_prefix=’/api’)
if __name__ == ‘__main__’:
app.run(host=’0.0.0.0′, debug=True, port=5050)
ChatGPT’s Response:
It even generated an updated version of the code that addresses some of the issues and listed what it changed.
5. Detecting Code Vulnerabilities
When writing code that contains sensitive information, whether it will be shipped to production or not, you have to be careful to avoid common anti-patterns that can result in vulnerabilities.
Here’s an example prompt and response to using ChatGPT to detect code vulnerabilities.
First, we tried asking ChatGPT to identify vulnerabilities in several code snippets, and we’re sharing this particular example below because it caught our attention. While we were aware of one major issue with the code’s assert statement, ChatGPT was still able to identify two additional vulnerabilities!
Prompt:
Is this code safe to run in a production environment?
from enum import Enum
from dataclasses import dataclass
class Role(Enum):
developer = “developer”
maintainer = “maintainer”
admin = “admin”
@dataclass
class User:
first_name: str
last_name: str
user_id: str
role: Role
def delete_table(table_id):
assert self.role == Role.admin.value
# implement deletion logic
pass
Chat GPT’s Response:
Not only did ChatGPT identify three relevant issues, but it also generated an updated version of the code that addresses the issues.
6. Providing an Optimized Implementation for a Code Snippet
You may encounter unexpected performance issues like high memory or CPU usage every time you push your code to production and run it. These issues are usually caused by poor designs or suboptimal codes. Fortunately, you can use tools like ChatGPT to optimize your function implementation and improve its efficiency.
Here’s an example prompt and response to using ChatGPT to provide an optimized implementation for a code snippet.
Prompt:
Can you give me an optimized version of this function?
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n-1) + fibonacci(n-2)
ChatGPT’s Response:
7. Generating Dummy Data
When the front end is ready before the API, it is a common practice for web developers to test the user interface (UI) with dummy data by hardcoding it. You can save a lot of time by using ChatGPT to generate dummy data with a specified format.
Here’s an example prompt and response to using ChatGPT to generate dummy data.
Prompt:
Generate an array of users with a unique id, name, and country.
ChatGPT’s Response:
8. Generating Data for Machine Learning Projects
Suppose you want to build an NLP model that sorts documents into one of the following categories: “news,” “sports,” and “entertainment.” You can ask ChatGPT to generate the type of data that you would use to train this model.
Here’s an example prompt and response to using ChatGPT to generate data for machine learning projects.
Prompt:
I want you to generate 20 sentences about these three classes, News, Sports, and Entertainment with the class name in front of each sentence.
ChatGPT’s Response:
This data can be programmatically retrieved if you connect to ChatGPT using this open-source package.
9. Generating Linux Commands
ChatGPT has been asked by many web developers to generate SQL commands, but in these examples, we will be asking it to generate Linux commands. This is because many web developers rely on them in their daily work and tend to forget some of the syntaxes.
Here’s an example prompt and response to using ChatGPT to generate Linux commands.
Prompt:
Generate a Linux command to unzip a tar.gz file.
ChatGPT’s Response:
Prompt:
Generate a Linux command to find all the files that match this pattern test_*.py. Copy these files inside a directory and tar it.
ChatGPT’s Response:
10. Detecting and Fixing Bugs
As a web developer, you spend more time debugging than writing code, and ChatGPT is also capable of debugging and repairing your code.
Here’s an example prompt and response to using ChatGPT to detect and fix bugs.
Prompt:
Is there any bug or error in my code? How can I fix it?
“`js
const username = “developeratul”
username = null
“`
ChatGPT’s Response:
The Bottom Line.
In conclusion, ChatGPT can be a game-changer for web developers looking to boost their productivity. With its advanced natural language processing capabilities and vast knowledge base, ChatGPT can provide quick and relevant solutions to a variety of coding and web development-related problems. Its ability to understand natural language and provide accurate and insightful responses can save developers time and effort in research and problem-solving, resulting in a more efficient workflow.
ChatGPT can also assist in brainstorming and ideation, by offering suggestions and ideas based on your queries. This can be particularly helpful for web developers looking to optimize their user interfaces or enhance the user experience of their websites. With ChatGPT, web developers can quickly access expert knowledge and advice. This makes it easier to stay up to date on the latest trends and best practices in web development.
Overall, ChatGPT can help web developers work smarter and more efficiently, allowing them to focus on high-value tasks and do more in less time. As this technology continues to evolve and improve, it’s likely that ChatGPT will become an indispensable tool for web developers around the world.
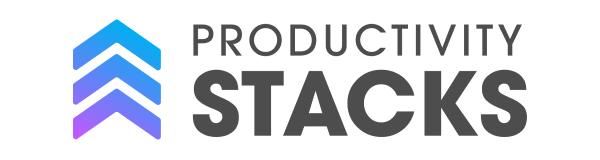